Thank you for your advice.
I try to your code.
Then I try first your code that send two value and I little change your code to send one value. i use both. result is below.
From your code I got some error.
and got this message from serial monitor
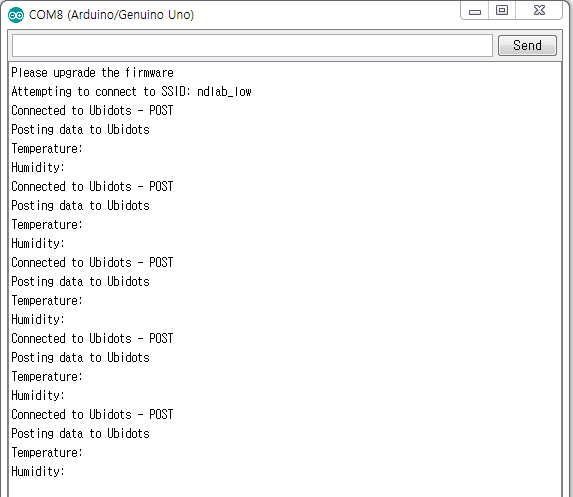
I don’t know why I got upgrade the firware message.actually i already done firmware upgrade. and I tested many Wi-Fi example.
and This time not got any result from both ubidots and Serial monitor.
Then I try send one value . make change little from your code.
I got same error like two value case (your code).
and This time not got a firmware upgrade message. but this case can’t see proper data from serial monitor.
you can see serial monitor data. and Also I can’t get a data from ubidots.
I used below code about one value case.
/********************************
* Libraries included
*******************************/
#include <WiFi.h>
#include <SPI.h>
/********************************
* Constants and objects
*******************************/
/* Assigns the network parameters */
char* WIFI_SSID = "a"; // your network WIFI_SSID (name)
char* WIFI_PASSWORD = "a"; // your network password
/* Assigns the Ubidots parameters */
char const * TOKEN = "a"; // Assign your Ubidots TOKEN
char const * DEVICE_LABEL = "arduino"; // Assign the unique device label
char const * VARIABLE_LABEL_1 = "temperature"; // Assign the unique variable label to publish data to Ubidots (1)
/* Parameters needed for the requests */
char const * USER_AGENT = "ARDUINO";
char const * VERSION = "1.0";
char const * SERVER = "things.ubidots.com";
int PORT = 80;
char topic[700];
char payload[300];
int status = WL_IDLE_STATUS;
/* initialize the library instance */
WiFiClient client;
/********************************
* Auxiliar Functions
*******************************/
void SendToUbidots(char* payload) {
int i = strlen(payload);
/* Builds the request POST - Please reference this link to know all the request's structures https://ubidots.com/docs/api/ */
sprintf(topic, "POST /api/v1.6/devices/%s/?force=true HTTP/1.1\r\n", DEVICE_LABEL);
sprintf(topic, "%sHost: things.ubidots.com\r\n", topic);
sprintf(topic, "%sUser-Agent: %s/%s\r\n", topic, USER_AGENT, VERSION);
sprintf(topic, "%sX-Auth-Token: %s\r\n", topic, TOKEN);
sprintf(topic, "%sConnection: close\r\n", topic);
sprintf(topic, "%sContent-Type: application/json\r\n", topic);
sprintf(topic, "%sContent-Length: %d\r\n\r\n", topic, i);
sprintf(topic, "%s%s\r\n", topic, payload);
/* Connecting the client */
client.connect(SERVER, PORT);
if (client.connected()) {
/* Sends the request to the client */
client.print(topic);
Serial.println("Connected to Ubidots - POST");
} else {
Serial.println("Connection Failed ubidots - Try Again");
}
/* Reads the response from the server */
while (client.available()) {
char c = client.read();
//Serial.print(c); // Uncomment this line to visualize the response on the Serial Monitor
}
/* Disconnecting the client */
client.stop();
}
/********************************
* Main Functions
*******************************/
void setup() {
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
/* Check for the presence of the shield */
if (WiFi.status() == WL_NO_SHIELD) {
Serial.println("WiFi shield not present");
/* don't continue */
while (true);
}
String fv = WiFi.firmwareVersion();
if (fv != "1.1.0") {
Serial.println("Please upgrade the firmware");
}
/* Attempt to connect to Wifi network */
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to SSID: ");
Serial.println(WIFI_SSID);
/* Connect to WPA/WPA2 network. Change this line if using open or WEP network */
status = WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
/* Wait 10 seconds for connection */
delay(10000);
}
}
void loop() {
/* Reads sensors values */
char* temperature = analogRead(A0);
String temp_value = String(temperature);
/* Builds the payload - {"temperature":25.00,"humidity":50.00} */
sprintf(payload, "{\"");
sprintf(payload, "%s%s\":%s", payload, VARIABLE_LABEL_1, temp_value.c_str());
sprintf(payload, "%s}", payload);
/* Calls the Ubidots Function POST */
SendToUbidots(payload);
/* Prints the data posted on the Serial Monitor */
Serial.println("Posting data to Ubidots");
Serial.print("Temperature: ");
Serial.println(temp_value);
delay(5000);
}